マイクロビット(m_13)モジュール gc love machine math
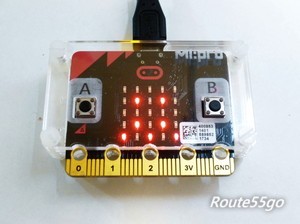
module – gc love machine math
thonny-microbitには「antigravity、array、audio、builtins、collections|ucollections、gc、love、machine、math、microbit、micropython、music、neopixel、os、radio、random、speech、sys、struct|ustruct、time|utime、this」のモジュールがあります。※microbitモジュールは既に記載しました。
(d-06)gc -module
garbage collectionとはプログラムで使っていないメモリを解放する機能のことらしいです。※よくわかりません。
gcモジュールには「collect, disable, enable, isenabled, mem_free, mem_alloc, threshold」の関数があります。
MicroPython ライブラリなどを参考にしました。詳細は、
「https://micropython-docs-ja.readthedocs.io/ja/latest/library/gc.html」
「https://docs.python.org/ja/3/library/gc.html」
を参照ください。
(06-1)gc.enable()
自動ガベージコレクションを有効にします。
>>> import gc
>>> gc.enable()
>>> gc.isenabled()
True
(06-2)gc.disable()
自動ガベージコレクションを無効にします。
無効にしてもヒープメモリの割り当ては可能で、gc.collect() を使って手動で開始できます。
gc.disable()するとメモリーエラーが発生し、STOPしないと続けてエラー発出しました。
>>> gc.isenabled()
True
>>> gc.disable()
>>> Traceback (most recent call last):
File "", line 1
MemoryError: parser could not allocate enough memory
(06-3)gc.collect()
ガベージコレクションを実行します。
>>> gc.mem_free()
5152
>>> gc.collect()
>>> gc.mem_free()
7808
(06-4)gc.isenabled()
自動ガベージコレクションが有効なら True を返します。
>>> import gc
>>> gc.isenabled()
True #初期から有効のようです。
(06-5)gc.mem_alloc()
割り当てられているヒープ RAM のバイト数を返します。
>>> import gc
>>> gc.mem_alloc()
5568
(06-6)gc.mem_free()
使用可能なヒープ RAM のバイト数を返します。この量がわからない場合は -1 を返します。
>>> import gc
>>> gc.mem_free()
1536
(06-7)gc.threshold([amount])
GC 割り当てしきい値を追加で設定または照会します。引数なしで関数を呼び出すと現在のしきい値を得ます。値が -1 の場合は無効な割り当てしきい値であることを意味します。 ※よくわかりません
以下では現在空いているヒープの25%以上が占有されるとGCが発生します。
>>> import gc
>>> gc.collect()
>>> gc.threshold(gc.mem_free()//4 + gc.mem_alloc())
>>>
(d-07)love -module
loveモジュールにはbadaboom()の関数があります。Image.HEARTが心臓のような点滅をします。
>>> from microbit import *
>>> import love #Image.HEARTが心臓的な点滅
>>> love
<module 'love'>
>>> love.badaboom() #Image.HEARTが心臓的な点滅
>>> display.show(Image.HEART)
(d-08)machine
micro:bit ハードウェアに関連する関数のモジュールです。
unique_id, reset, freq, disable_irq, enable_irq, mem8, mem16, mem32, time_pulse_usがあります。
(08-1)machine.unique_id
ボードの識別子をバイト列を返します。ボードの個体ごとに異なります。
>>> import machine
>>> machine.unique_id()
b'\xe4!@\x85i2 \x8a'
(08-2)machine.reset()
外部 RESET ボタンを押すのと同じようにデバイスをリセットします。
>>> import machine
>>> machine.reset()
MicroPython v1.9.2-34-gd64154c73 on 2017-09-01; micro:bit v1.0.1 with nRF51822
Type "help()" for more information.
>>>
(08-3)machine.freq()
CPU周波数をヘルツ単位で返します。
>>> import machine
>>> machine.freq()
16000000 #16MHz
>>>
(08-4)machine.disable_irq()
割り込みを禁止にします。状態の記憶が必要です。
(08-5)machine.enable_irq()
割り込みを可能にします。割り込み禁止した状態値を戻す必要があります。
#!/usr/bin/env python
# -*- coding: utf-8 -*-
from microbit import *
import machine
state = machine.disable_irq() #割り込み禁止 state(状態)を記録していく
print('irq_disable=',state)
for i in range(5):
print(i)
sleep(1000)
machine.enable_irq(state) #割り込み許可時に state(状態)を指定する
print('irq_enable')
普通に実行した場合
>>> %Run 1005_irq_01.py
irq_disable= 0
0
1
2
3
4
irq_enable
>>>
0~4が表示される間にCtrl+Cで割り込みしても、割り込みを禁止しているので停止しません。
割り込みが許可になってから停止します。
ちなみに割り込み禁止が無いただの繰返しだけの場合(for~printの9~11行だけ)では、2でCtrl+Cすると即停止します。
>>> %Run 1005_irq_01.py
0
1
Traceback (most recent call last):
File "C:\Py_B551\02tes\1005_irq_01.py", line 11, in
KeyboardInterrupt:
>>>
(08-6)machine.time_pulse_us(pin, pulse_level, timeout_us=1000000)
指定の pin のパルス時間をマイクロ秒単位で返します。
pulse_level
Lowレベル測定(toff)には0、High測定(ton)には1を指定します。タイムアウト時間は timeout_us (マイクロ秒単位)で指定します。
測定開始時のピン入力値がpulse_level に等しい場合、計測はすぐに開始されます。pulse_level と異なる場合、ピン入力が pulse_level になるまで待機しpulse_level になれば測定します。その際、待機中でタイムアウトが発生した場合 -2 を返し、測定中でタイムアウトが発生した場合は -1 を返します。
※pin0とpin1は直接接続しています。
#!/usr/bin/env python
# -*- coding: utf-8 -*-
from microbit import *
import machine
pin0.set_pull(pin0.PULL_DOWN) #一応 プル設定をしておく
pin1.write_analog(0) #アナログ出力>周期設定>パルス幅設定
pin1.set_analog_period_microseconds(500) #2kHz 500usec
pin1.write_analog(128) #500/1024*128=63us
while True:
d = machine.time_pulse_us(pin0,1) # Ton時間測定
sleep(500)
print(d)
測定値の表示>だいたい合ってる。
>>> %Run 1005_time_pulse_1.py
65
64
65
65
65
65
64
65
========================= RESTART =========================
出力波形 T=500usec、write_analog(128)
(08-7)machine.mem
mem8
mem16
mem32
物理アドレスを指定してデバイスのメモリから、
1バイト(8ビット; mem8)、
2バイト(16ビット; mem16)、
4バイト(32ビット; mem32)のワードを取得できます。
たとえば mem8[0x00] は、物理アドレス 0x00 の1バイトを読み取ります。
>>> import machine
>>>
>>> a=machine.mem16[0x00]
>>> a
16384
>>> type(a)
<class 'int'>
>>> hex(a)
'0x4000'
(d-09)math-module
mathモジュールを読み込むと算術関数が使えます。「e, pi, sqrt, pow, exp, log, cos, sin, tan, acos, asin, atan, atan2, ceil, copysign, fabs, floor, fmod, frexp, ldexp, modf, isfinite, isinf, isnan, trunc, radians, degrees」の関数があります。
※詳細はPythonのドキュメントサイトなどを参照してください。
>>> import math
>>> deg=45
>>> rad=math.radians(deg)
>>> math.tan(rad)
1.0
>>> math.sin(rad)
0.707107
>>> 1/math.sqrt(2)
0.707107
まとめ
thonny-microbitのモジュール gc、love、machine、math について記載しました。